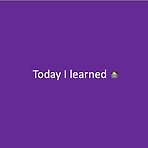
Module 1. app.Module생성 $ nest g moimport { Module } from '@nestjs/common'; import { MoviesModule } from './movies/movies.module'; import { AppController } from './app.controller'; //앱을 만들 때 모듈로 분리하면 좋다. 기존에 있던 MovieService와 MovieController를 따로 movies.module.ts를 생성하여, 넣어주었다. @Module({ imports: [MoviesModule], controllers: [AppController], providers: [], }) export class App..
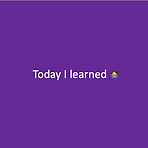
Validation movieData와 updateData의 데이터 타입에 따른 예외처리 1. movieData DTO(데이터 전송 객체 , Data Transfer Object) 생성 dto 폴더 생성 및 create-movie.dto.ts 파일생성하여 dto 타입을 지정해준다. // 클라이언트가 보낼수 있는 전송객체 export class CreateMovieDto { readonly title: string; readonly year: number; readonly genres: string[]; } 유효성 검사 1 : ValidationPipe() main.ts에 ValidationPipe추가 app.useGlobalPipes(new ValidationPipe()); import { Validat..
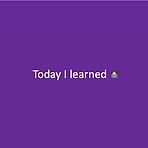
NestJS_REST_API_Service 우리는 Single-responsibility principle에 따라 코드를 작성할 예정이다. Single-responsibility principle란? 하나의 module, class 혹은 fuction이 하나의 기능은 꼭 책임져야한다는 뜻이다. 1. Service 생성 nest g s 2. service에서 사용할 데이터베이스를 생성한다. entity 폴더를 만들어 movie.entity.ts 파일을 생성하여 데이터의 모델을 지정해준다. export class Movie { id: number; title:string; year:number; genres: string[]; }3. movie.service.ts 작성 import { Injectable, ..
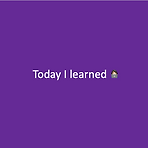
NestJS REST API 이번 블로그 부터는 NestJS를 사용하여 영화 관련 API를 만들어보겠습니다. 오늘은 그 시작의 첫번째, Controller입니다. 1. controller 생성 nest cli를 사용하여, controller를 생성해보겠습니다. 아래와 같이 nest cli가 지원하는 명령어가 있으며, nest generate controller 의 약자로 아래의 명령어를 실행해주고, nest g co '컨트롤러명' 을 적어줍니다. 놀라운 것은!!! movie 이름을 가진 폴더안에 컨트롤러가 자동 생성되고 동시에 module에 import가 자동으로 되었다!!! Amazing!!!!!!!! import { Module } from '@nestjs/common'; import { Movies..
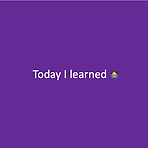
NestJS란? 효율적이고 확장 가능한 Node.js 서버 측 애플리케이션을 구축하기위한 프레임 워크. 즉, Node.js 위에서 움직이는 프레임워크 다른 node.js프레임워크에 없는 것을 가지고 있다. node.js에서는 url, controller, templete, middle ware 등을 어느 위치에 두든 상관 없이 돌아가게 할 수 있으나, Nest.js에서는 구조와 규칙이 정해져 있다. 객체지향 프로그래밍과 함수형 프로그래밍, 심지어는 함수 반응형 프로그래밍의 요소도 일부분 사용하는 것을 알 수 있다. ** NestJS 공식 홈페이지 : https://docs.nestjs.com/ [Documentation | NestJS - A progressive Node.js framework Nest..
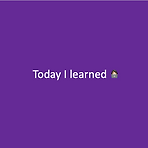
Interface 만들기 typscript interface Human { name : string; age : number; gender: string; } const person = { name : "Melody", age : 29, gender: "Female" } const sayHi = (person : Human):string => { return `hi, I'm ${person.name}, ${person.gender}, ${person.age}`; }; console.log(sayHi(person)); export {};typscript -> javastript "use strict"; Object.defineProperty(exports, "__esModule", { value: ..
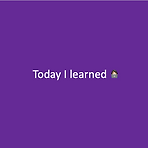
index.ts 작성시 const name1 = "Melody", age1 = 29, gender1 = "Female"; const sayHi = (name, age, gender?) :void => { // void : 빈공간이라는 뜻 return 값이 없을 때 사용 console.log(`hi, I'm ${name}, ${gender}, ${age}`) }; // sayHi(name1, age1, gender1); sayHi("melody", 29, "Female"); export {}; //export를 설정하지 않으면, name이라는 변수가 다른곳에 선언되었다고 오류가 뜬다. // 오류가 뜬다.모듈인 것을 이해할 수 있도록 export 작성 // cf. return 값이 String 인 경..
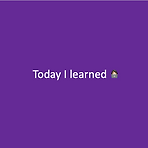
PostgreSQL Setting 설치 $ brew install postgresql 서비스 시작 $ brew services start postgresql psql 접속 $ psql postgres $ psql postgres -U melody psql 데이터베이스 생성 postgres=# CREATE DATABASE "test_db" WITH OWNER = test ENCODING = 'UTF8' template = template0; 데이터베이스 리스트 보기 postgres=> \list 테이블 리스트 보기 postgres=> \dt 특정 database로 연결하기 postgres=> \connect test postgres=# 가 postgres=> 로 바뀐 것을 확인 할 수 있음. 특정 유저에게..
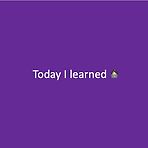
Typescript 왜 써야할까!?? Typescript의 장점은 먼저 type에대해 유연한 Javascript와 다르게 컴파일 단계에서 타입을 체크해주므로 Type의 오류를 실행 전단계에서 미리 점검하고 갈수있는 Type Safe Code 를 작성할수 있는 점이 있다. Typescript 의 특징 객체지향 프로그래밍 ES6클래스 Interface 지원 : 클래스와 객체를 구조화 클래스 접근제한자 지원(public, protected, private) : 은닉성, 캡슐화와 같은 OOP를 구현하는데에 도움을 줌. 따라서 2020트렌드는 TypeScript라 할 수 있겠다. VSC code에서 Typescript 사용하기 👉 TSlint 설치 TypeScrit 시작하기 nvm 버전을 8.x.x 이상으로 설정..