View
문제
정렬된 두 묶음의 숫자 카드가 있다고 하자. 각 묶음의 카드의 수를 A, B라 하면 보통 두 묶음을 합쳐서 하나로 만드는 데에는 A+B 번의 비교를 해야 한다. 이를테면, 20장의 숫자 카드 묶음과 30장의 숫자 카드 묶음을 합치려면 50번의 비교가 필요하다.
매우 많은 숫자 카드 묶음이 책상 위에 놓여 있다. 이들을 두 묶음씩 골라 서로 합쳐나간다면, 고르는 순서에 따라서 비교 횟수가 매우 달라진다. 예를 들어 10장, 20장, 40장의 묶음이 있다면 10장과 20장을 합친 뒤, 합친 30장 묶음과 40장을 합친다면 (10 + 20) + (30 + 40) = 100번의 비교가 필요하다. 그러나 10장과 40장을 합친 뒤, 합친 50장 묶음과 20장을 합친다면 (10 + 40) + (50 + 20) = 120 번의 비교가 필요하므로 덜 효율적인 방법이다.
N개의 숫자 카드 묶음의 각각의 크기가 주어질 때, 최소한 몇 번의 비교가 필요한지를 구하는 프로그램을 작성하시오.
입력
첫째 줄에 N이 주어진다. (1 ≤ N ≤ 100,000) 이어서 N개의 줄에 걸쳐 숫자 카드 묶음의 각각의 크기가 주어진다. 숫자 카드 묶음의 크기는 1,000보다 작거나 같은 양의 정수이다.
출력
첫째 줄에 최소 비교 횟수를 출력한다.
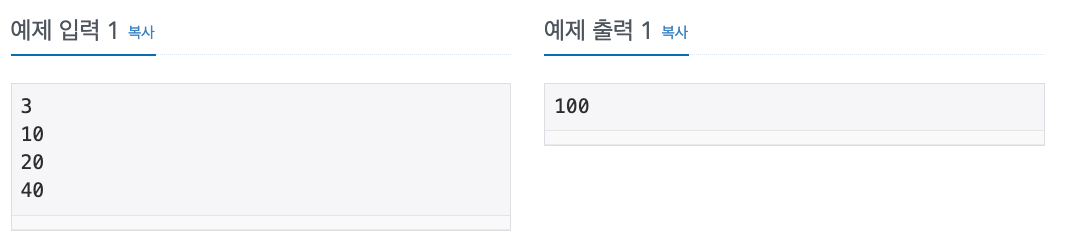
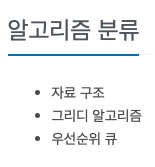
나의 풀이
1 . 출력초과나온 오답 - deque
# 09:40 ~ 10:10
import sys
from collections import deque
input = sys.stdin.readline
n = int(input())
num_list = deque([])
for _ in range(n):
card_cnt = int(input())
num_list.append(card_cnt)
if len(num_list) > 1:
a = sorted(list(num_list))
num_sort = deque(a)
init = num_sort.popleft() + num_sort.popleft()
while num_sort:
item = num_sort.popleft()
init = init * 2 + item
print(init)
else:
print(0)
2. 정답 - heapq (우선순위큐)
# 10:10 - 11:30
import sys
import heapq
input = sys.stdin.readline
num_list = []
for _ in range(int(input())):
card_cnt = int(input())
heapq.heappush(num_list, card_cnt)
if len(num_list) > 1:
result = 0
while len(num_list)>1:
item = heapq.heappop(num_list) +heapq.heappop(num_list)
result += item
heapq.heappush(num_list, item)
print(result)
else:
print(0)
3. 정답 - PriorityQueue(우선순위큐)
import sys
from queue import PriorityQueue
num_list = PriorityQueue()
input = sys.stdin.readline
for _ in range(int(input())):
num_list.put(int(input()))
if num_list.qsize() > 1:
result = 0
while num_list.qsize()>1:
item = num_list.get() + num_list.get()
result += item
num_list.put(item)
print(result)
else:
print(0)
heqpq
최소값이나 최대값을 빨리 찾아야 할 때
최소 힙
최대힙
Heaqp와 PriorityQueue의 성능비교
테스트 결과 Heaqp를 사용한 우선순위 큐 구현이 더 성능이 우수함을 알 수 있었다.
- 메모리 : Heaqp ( 33796 KB ) < PriorityQueue(38064 KB)
- 시간 : Heaqp ( 176 ms) < PriorityQueue(564 ms)
Reference
'코딩테스트 > 백준' 카테고리의 다른 글
BJ_1439) [그리디] 뒤집기 (0) | 2022.12.21 |
---|---|
BJ_11652) 카드 (0) | 2022.12.20 |
BJ_11724) 연결요소의 개수 (0) | 2022.12.19 |
BJ_1946) 신입사원 (0) | 2022.12.19 |
BJ_10610) 30 (0) | 2022.12.17 |
reply